following is very simple approach, how to do that-
1) You need to add following section in your Web.config
<system.web>
<urlMappings enabled="true">
<add url="~/fakename.aspx" mappedUrl="~/actualpageurl.aspx" />
<add url="~/Registration.aspx" mappedUrl="~/signup.aspx" />
</urlMappings>
</system.web>
2) Add ASp.Net folder "App_Browsers" in your application
3) Add a new item in that folder as "Form.browser"
Code- Form.browser
<browsers>
<browser refID="Default">
<controlAdapters>
<adapter controlType="System.Web.UI.HtmlControls.HtmlForm"
adapterType="FormRewriterControlAdapter" />
</controlAdapters>
</browser>
</browsers>
4) Create a class file as follows
//Form.cs
using System;
using System.Web.UI;
using System.Web;
public class FormRewriterControlAdapter : System.Web.UI.Adapters.ControlAdapter
{
protected override void Render(System.Web.UI.HtmlTextWriter writer)
{
base.Render(new RewriteFormHtmlTextWriter(writer));
}
}
public class RewriteFormHtmlTextWriter : HtmlTextWriter
{
public RewriteFormHtmlTextWriter(HtmlTextWriter writer)
: base(writer)
{
this.InnerWriter = writer.InnerWriter;
}
public RewriteFormHtmlTextWriter(System.IO.TextWriter writer)
: base(writer)
{
base.InnerWriter = writer;
}
public override void WriteAttribute(string name, string value, bool fEncode)
{
// If the attribute we are writing is the "action" attribute, and we are not on a sub-control,
// then replace the value to write with the raw URL of the request - which ensures that we'll
// preserve the PathInfo value on postback scenarios
if ((name == "action"))
{
HttpContext Context = default(HttpContext);
Context = HttpContext.Current;
if (Context.Items["ActionAlreadyWritten"] == null)
{
// Because we are using the UrlRewriting.net HttpModule, we will use the
// Request.RawUrl property within ASP.NET to retrieve the origional URL
// before it was re-written. You'll want to change the line of code below
// if you use a different URL rewriting implementation.
value = Context.Request.RawUrl;
// Indicate that we've already rewritten the <form>'s action attribute to prevent
// us from rewriting a sub-control under the <form> control
Context.Items["ActionAlreadyWritten"] = true;
}
}
base.WriteAttribute(name, value, fEncode);
}
}
5) Thats all and you are ready to rewrite your incoming URLs without any complex code for HTTP-module
and any actionless form.
IMPORTANT: If you go for Actionless form then it has a side effect that disables page validators.
if this was helpful then please donate as much you want
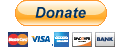
Cheers
12 comments:
Any idea how to achieve this in Sharepoint . Means where to add this browser tag if I want the same functionality for built in links in sharepoint.
Hey I'd like to congratulate you for such a terrific quality forum!
I was sure this would be a nice way to introduce myself!
Sincerely,
Edwyn Sammy
if you're ever bored check out my site!
[url=http://www.partyopedia.com/articles/tea-party-supplies.html]tea Party Supplies[/url].
this is the best answer to this:
[http://webgeek.elletis.com/difference-between-url-mapping-and-url-rewriting/]
you may find this article useful:
http://webgeek.elletis.com/difference-between-url-mapping-and-url-rewriting/
i was looking for like evrywhere now i will implement it and tell you how it goes aethtech
Hello Dear,
I like you blog very much, it's a really use full blog and i read daily.
You can also find Article Rewriting Service with us.
Are You Looking for an article rewriter? look no further, our Article Rewriting Service offers human based article writing services to business at CopyPeer.
Really nice blog post.provided a helpful information.I hope that you will post more updates like this
.NET Online Training
world777 betting
world777 agent
aws solution architect training
azure solution architect certification
openshift certification
azure data engineer certification
ebs on oci free class
azure sa exam questions
aws sa free training
aws sa interview questions
aws solutions architect exam questions
aws sa free class
da-100 exam questions
da100 free class
docker free training
cka free training
Post a Comment